MySQL Commands
//select database
mysql> use [databasename]
//show all databases
mysql> show databases;
//show database tables
mysql> show tables;
//describe table, must have the database selected
mysql> show tables;
//a search query that allow regular expressions
SELECT * FROM customers WHERE city like 'Air%';
//create table, must have a type after the name. eg: name=customerid, type=text
create table books
(customerid text, name text);
//delete table
drop table balls;
//insert values
insert into customers values
(null,'blahblah', 3);
//connecting subqueries
select name from customers where customers.customerid = any (select orders.orderid from orders,order_items where orders.orderid = order_items.orderid);
//Update record
update books set price = price1.1;
//alter table, add columns. its like create table, name and type
alter table books
add (tax text);
alter table books
drop tax;
//delete record
delete from customers
where cus
Wednesday, August 31, 2011
Monday, August 29, 2011
Center HTML/Webpage
To center your webpage its simple. By setting the width and margin you can achieve this. However, depending on the browser (Internet Explorer) you must include the doctype or it will not work. See the code below
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<body>
<html>
<style>
#main {
width: 900px;
margin: 0 auto;
}
</style>
</head>
<body>
<div id="main">
</div>
</body>
</html>
Thursday, August 25, 2011
Quote: Stick to it
Steve Jobs stepped down as CEO at Apple today. He is an inspiration to me and many others through his products, life and beliefs.
The problem with the Internet startup craze isn’t that too many people are starting companies; it’s that too many people aren’t sticking with it. That’s somewhat understandable, because there are many moments that are filled with despair and agony, when you have to fire people and cancel things and deal with very difficult situations. That’s when you find out who you are and what your values are.
So when these people sell out, even though they get fabulously rich, they’re gypping themselves out of one of the potentially most rewarding experiences of their unfolding lives. Without it, they may never know their values or how to keep their newfound wealth in perspective. -Steve Jobs
The problem with the Internet startup craze isn’t that too many people are starting companies; it’s that too many people aren’t sticking with it. That’s somewhat understandable, because there are many moments that are filled with despair and agony, when you have to fire people and cancel things and deal with very difficult situations. That’s when you find out who you are and what your values are.
So when these people sell out, even though they get fabulously rich, they’re gypping themselves out of one of the potentially most rewarding experiences of their unfolding lives. Without it, they may never know their values or how to keep their newfound wealth in perspective. -Steve Jobs
Wednesday, August 24, 2011
Frameworks
Don't reinvent the wheel. Below are frameworks that allow you to code without having to start from scratch.
HTML - Boilerplate
PHP - CodeIgniter
CSS - Twitter Bootsrap
CSS structure - 960
Javascript - jQuery
HTML - Boilerplate
PHP - CodeIgniter
CSS - Twitter Bootsrap
CSS structure - 960
Javascript - jQuery
Sunday, August 21, 2011
Self-discipline
Simple definition to self-discipline
The ability to make yourself do, what you know you should do, when you should do it, when you feel like it or not. That's the difference between success and failer is whether you feel like it or not. -Brian Tracy
The ability to make yourself do, what you know you should do, when you should do it, when you feel like it or not. That's the difference between success and failer is whether you feel like it or not. -Brian Tracy
Saturday, August 20, 2011
Giveaway
This is baligena first Giveaway. What better way to thank all you who been supporting the site. baligena will be giving an iPod shuffle. The giveaway starts today and ends on September 3rd 2011.
Now here is how to win
1. First you must subscribe to my feed by putting your email under "Follow by Email" on the left sidebar and click submit.
2. Leave a comment on any of my post saying "This is the first comment".
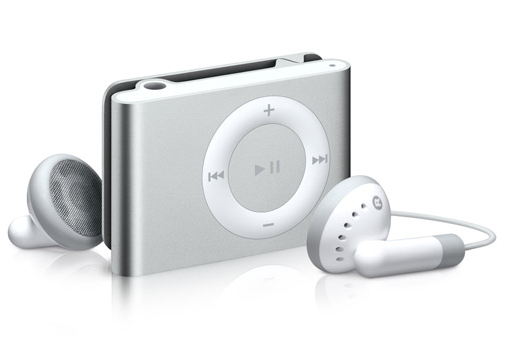
Now here is how to win
1. First you must subscribe to my feed by putting your email under "Follow by Email" on the left sidebar and click submit.
2. Leave a comment on any of my post saying "This is the first comment".
Wednesday, August 17, 2011
Monday, August 15, 2011
Principle of Least Privilege
The principle of least privilege can be used to improve the security of any computer system. It's a basic but important principle that is often overlooked. The principle is as follows:
A user (or process) should have the lowest level of privilege required to perform his assigned task.
It applies in MySQL as it does elsewhere. For example, to run queries from the Web, a user does not need all the privileges to which "root" has access. You should therefore create another user who has only the necessary privileges to access the database you just created.
Excerpt from PHP and MySQL Web Development page 223
A user (or process) should have the lowest level of privilege required to perform his assigned task.
It applies in MySQL as it does elsewhere. For example, to run queries from the Web, a user does not need all the privileges to which "root" has access. You should therefore create another user who has only the necessary privileges to access the database you just created.
Excerpt from PHP and MySQL Web Development page 223
Friday, August 12, 2011
Quote: The climb is the best part
"To climb up the mountain is the best thing. you know preparing for it. Living every day in practice, working hard, talking about it, seeing the commitment out of the kids, everybody on the team is at practice, 100 percent all the time and just working hard every day. That’s where the comradely happens that’s where you bond and hopefully when you get the chance you'll be able to do it" -Russ Cozart Brandon High School Wrestling Coach
Cozart makes this statement in an interview about the next chance he gets at the National High School Dual Meet Wrestling Tournament after lost in the finals.
Watch the interview
Cozart makes this statement in an interview about the next chance he gets at the National High School Dual Meet Wrestling Tournament after lost in the finals.
Watch the interview
Wednesday, August 10, 2011
Youtube Data API
Below is a PHP script that uses Youtube API through xml to show the comments on your personal website.
I didn't include any CSS.
Use this link a reference
http://code.google.com/intl/it-IT/apis/youtube/2.0/developers_guide_protocol.html
}
?>
I didn't include any CSS.
Use this link a reference
http://code.google.com/intl/it-IT/apis/youtube/2.0/developers_guide_protocol.html
<?php';
//// video id
$videoId='M1Wm9nGJcYo';
//echo the video
echo "";
// url where feed is located
$url="http://gdata.youtube.com/feeds/api/videos/$videoId/comments";
// get the feed
$comments=simplexml_load_file($url);
// parse the feed using a loop
foreach($comments->entry as $comment)
{
echo '
}
?>
Monday, August 8, 2011
.inc vs .php file extention
.inc stands for include.
Some may prefer to name their file page.inc when using the php function include() because it looks prettier.
Some may prefer to name their file page.inc when using the php function include() because it looks prettier.
include('page.inc');
page.inc or page.php both work the same way.
Saturday, August 6, 2011
Sending Text Message with PHP
Below are resources on sending text messages with PHP.
http://net.tutsplus.com/tutorials/php/how-to-send-text-messages-with-php/
http://www.venture-ware.com/kevin/web-development/email-to-sms/
https://www.tropo.com/
http://net.tutsplus.com/tutorials/php/how-to-send-text-messages-with-php/
http://www.venture-ware.com/kevin/web-development/email-to-sms/
https://www.tropo.com/
Thursday, August 4, 2011
Quote: Taking risk
Life is about taking risks. You are very young and have a promising future. Why are you willing to subject yourself to this disability just because of that 1% risk? Take that 99% chance of success and do yourself a favor."
Monday, August 1, 2011
AutoStart WAMP when windows start(apache and mysql)
Auto starting Mysql and Apache when windows starts gives you a desktop application feel built on PHP.
The Instructions:
Easiest way is to move 'start wampserver' from the start>program menu>wampserver and drag it into your start>program menu>startup folder. However if windows ask for permission to start the program the option below will fit your needs better
wampapache
wampmysql
The Instructions:
Easiest way is to move 'start wampserver' from the start>program menu>wampserver and drag it into your start>program menu>startup folder. However if windows ask for permission to start the program the option below will fit your needs better
Go start>run> type in 'services.msc' and press enter
scroll down to the two serviceswampapache
wampmysql
right click them and select properties.
on the general tab change startup from manual to automatic.WARNING: Doing this method will not show the wamp icon at the bottom right hand conner of screen
in order to adjust Apache and Mysql settings start wamp and the icon will then appear
Subscribe to:
Posts (Atom)